CSE LABS
Labs for I Year
Program Objectives
- Understand the basic concept of C Programming, and its different modules that include
- Conditional and looping expressions, Arrays, Strings, Functions, Pointers, and Structures.
- Acquire knowledge about the basic concept of writing a program.
- Role of constants, variables, identifiers, operators, type conversion and other building blocks of C Language.
- Use of conditional expressions and looping statements to solve problems associated with conditions and repetitions.
- Role of Functions involving the idea of modularity.
- Programming using gcc compiler in Linux.
List of Experiments
Practice Sessions
- Write a simple program that prints the results of all the operators available in C (including pre/ post increment , bitwise and/or/not , etc.). Read required operand values from standard input.
- Write a simple program that converts one given data type to another using auto conversion and casting. Take the values form standard input.
Simple Numeric Problems
- Write a program for fiend the max and min from the three numbers.
- Write the program for the simple, compound interest.
- Write program that declares Class awarded for a given percentage of marks, where mark = 70% = Distinction. Read percentage from standard input.
- Write a program that prints a multiplication table for a given number and the number of rows in the table. For example, for a number 5 and rows = 3, the output should be:
- 5 x 1 = 5
- 5 x 2 = 10
- 5 x 3 = 15
- Write a program that shows the binary equivalent of a given positive number between 0 to 255
Expression Evaluation
- A building has 10 floors with a floor height of 3 meters each. A ball is dropped from the top of the building. Find the time taken by the ball to reach each floor. (Use the formula s = ut+(1/2)at^2 where u and a are the initial velocity in m/sec (= 0) and acceleration in m/sec^2 (= 9.8 m/s^2)).
- Write a C program, which takes two integer operands and one operator from the user, performs the operation and then prints the result. (Consider the operators +,-,*, /, % and use Switch Statement)
- Write a program that finds if a given number is a prime number
- Write a C program to find the sum of individual digits of a positive integer and test given number is palindrome.
- A Fibonacci sequence is defined as follows: the first and second terms in the sequence are 0 and 1. Subsequent terms are found by adding the preceding two terms in the sequence. Write a C program to generate the first n terms of the sequence.
- Write a C program to generate all the prime numbers between 1 and n, where n is a value supplied by the user.
- Write a C program to find the roots of a Quadratic equation.
- Write a C program to calculate the following, where x is a fractional value.
- 1-x/2 +x^2/4-x^3/6
- Write a C program to read in two numbers, x and n, and then compute the sum of this geometric progression: 1+x+x^2+x^3+………….+x^n. For example: if n is 3 and x is 5, then the program computes 1+5+25+125.
Arrays and Pointers and Functions
- Write a C program to find the minimum, maximum and average in an array of integers.
- Write a functions to compute mean, variance, Standard Deviation, sorting of n elements in single dimension array.
- Write a C program that uses functions to perform the following
- Addition of Two Matrices
ii. Multiplication of Two Matrices
iii. Transpose of a matrix with memory dynamically allocated for the new matrix as row and column counts may not be same. - Write C programs that use both recursive and non-recursive functions
- To find the factorial of a given integer
ii. To find the GCD (greatest common divisor) of two given integers.
iii. To find x^n k. Write a program for reading elements using pointer into array and display the values using array. - Write a program for display values reverse order from array using pointer.
- Write a program through pointer variable to sum of
- elements from array
Files
- Write a C program to display the contents of a file to standard output device.
- Write a C program which copies one file to another, replacing all lowercase characters with their uppercase equivalents.
- Write a C program to count the number of times a character occurs in a text file. The file name and the character are supplied as command line arguments.
- Write a C program that does the following: It should first create a binary file and store 10 integers, where the file name and 10 values are given in the command line. (hint: convert the strings using atoi function) Now the program asks for an index and a value from the user and the value at that index should be changed to the new value in the file. (hint: use fseek function) R18 B.Tech. CSE Syllabus JNTU HYDERABAD 28 The program should then read all 10 values and print them back.
- Write a C program to merge two files into a third file (i.e., the contents of the firs t file followed by those of the second are put in the third file).
Strings
- Write a C program to convert a Roman numeral ranging from I to L to its decimal equivalent
- Write a C program that converts a number ranging from 1 to 50 to Roman equivalent
- Write a C program that uses functions to perform the following operations
i. To insert a sub-string in to a given main string from a given position.
ii. To delete n Characters from a given position in a given string. - Write a C program to determine if the given string is a palindrome or not (Spelled same in both directions with or without a meaning like madam, civic, noon, abcba, etc.)
- Write a C program that displays the position of a character ch in the string S or – 1 if S doesn‘t contain ch.
- Write a C program to count the lines, words and characters in a given text.
Miscellaneous
- Write a menu driven C program that allows a user to enter n numbers and then choose between finding the smallest, largest, sum, or average. The menu and all the choices are to be functions. Use a switch statement to determine what action to take. Display an error message if an invalid choice is entered.
- Write a C program to construct a pyramid of numbers as follows
1 . 1 1 .
1 2 . . 2 3 2 2 . .
1 2 3 . . . 4 5 6 3 3 3 . . .
4 4 4 . .
Sorting and Searching
- Write a C program that uses non recursive function to search for a Key value in a given
- list of integers using linear search method.
- Write a C program that uses non recursive function to search for a Key value in a given
- Sorted list of integers using binary search method.
- Write a C program that implements the Bubble sort method to sort a given list of
- integers in ascending order.
- Write a C program that sorts the given array of integers using selection sort in descending order
- Write a C program that sorts the given array of integers using insertion sort in ascending order
- Write a C program that sorts a given array of names
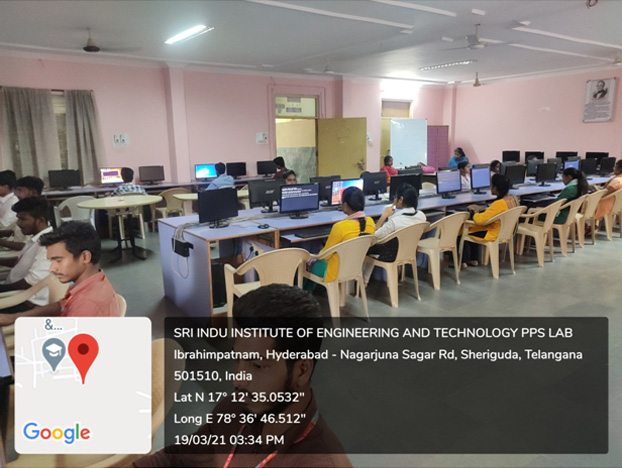
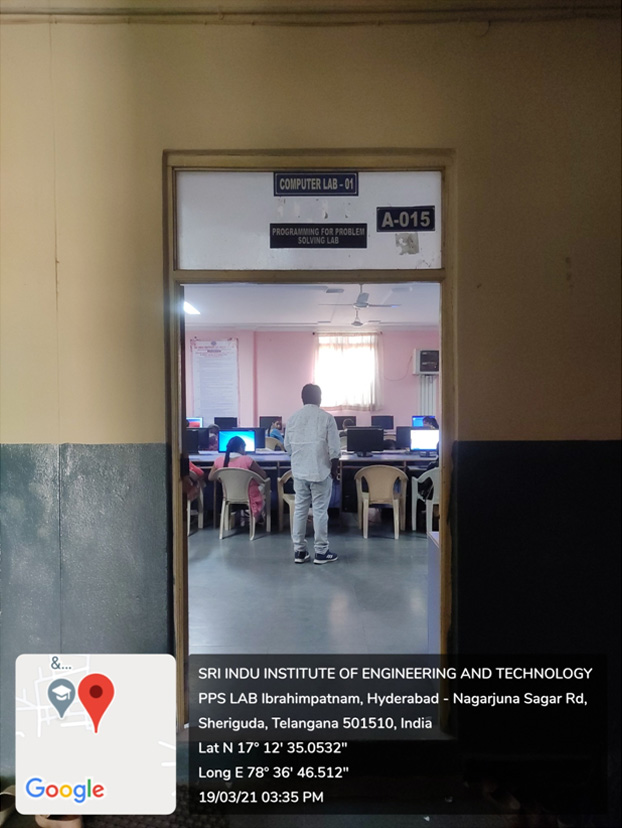
Labs for II Year I Semester
- A C++ program is a collection of program files (at least one) and header files (zero or more) containing functions and classes.
- This collection of functions and classes making up a program is called the source code for that program.
- Program files typically have the extension
List of Experiments
- Write a C++ Program to display Names, Roll No., and grades of 3 students who have appeared in the examination. Declare the class of name, Roll No. and grade. Create an array of class objects. Read and display the contents of the array.
- Write a C++ program to declare Struct. Initialize and display contents of member variables.
- Write a C++ program to declare a class. Declare pointer to class. Initialize and display the contents of the class member.
- Given that an EMPLOYEE class contains following members: data members:
Employee number, Employee name, Basic, DA, IT, Net Salary and print data members - Write a C++ program to read the data of N employee and compute Net salary of each employee (DA=52% of Basic and Income Tax (IT) =30% of the gross salary).
- Write a C++ to illustrate the concepts of console I/O operations.
- Write a C++ program to use scope resolution operator. Display the various values of the same variables declared at different scope levels.
- Write a C++ program to allocate memory using new operator.
- Write a C++ program to create multilevel inheritance. (Hint: Classes A1, A2, A3)
- Write a C++ program to create an array of pointers. Invoke functions using array objects.
- Write a C++ program to use pointer for both base and derived classes and call the member function. Use Virtual keyword.
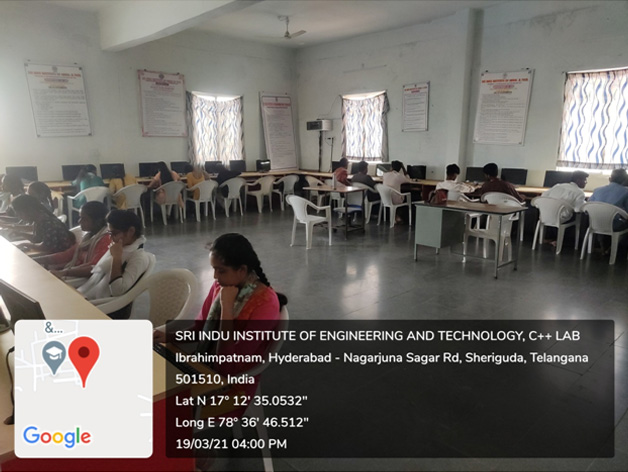
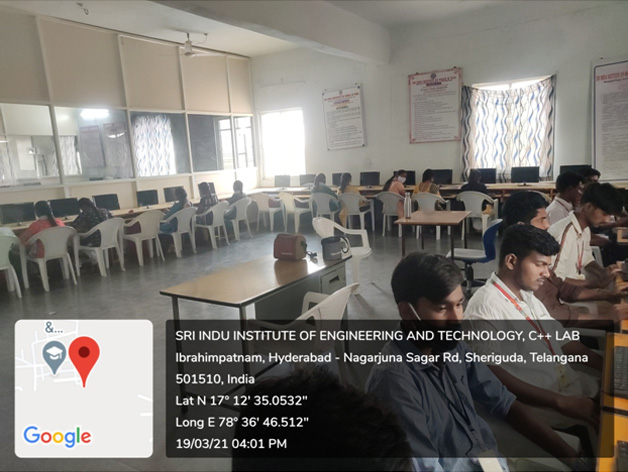
- Course Objectives: It covers various concepts of C programming language
- It introduces searching and sorting algorithms
- It provides an understanding of data structures such as stacks and queues
- Course Outcomes: Ability to develop C programs for computing and real-life applications using basic elements like
- Control statements, arrays, functions, pointers and strings, and data structures like stacks, queues and linked lists. Ability to Implement searching and sorting algorithms
List of Experiments
- Write a program that uses functions to perform the following operations on singly linked list.:
i) Creation ii) Insertion iii) Deletion iv) Traversal - Write a program that uses functions to perform the following operations on doubly linked list.:
i) Creation ii) Insertion iii) Deletion iv) Traversal - Write a program that uses functions to perform the following operations on circular linked list.:
i) Creation ii) Insertion iii) Deletion iv) Traversal - Write a program that implement stack (its operations) using
i) Arrays ii) Pointers - Write a program that implement Queue (its operations) using
i) Arrays ii) Pointers - Write a program that implements the following sorting methods to sort a given list of integers in ascending order
i) Bubble sort ii) Selection sort iii) Insertion sort - Write a program that use both recursive and non recursive functions to perform the following searching operations for a Key value in a given list of integers:
i) Linear search ii) Binary search - Write a program to implement the tree traversal methods.
- Write a program to implement the graph traversal methods
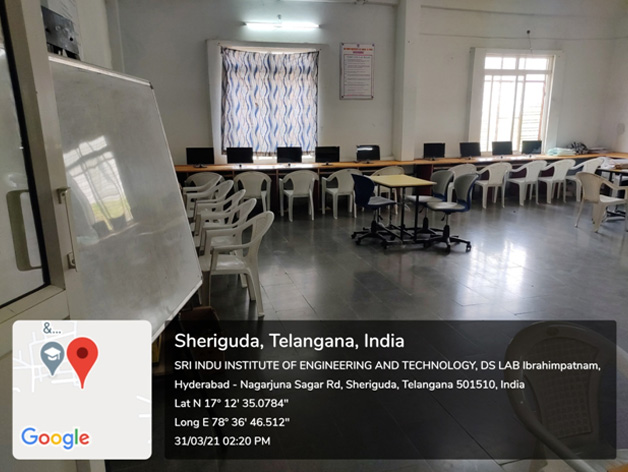
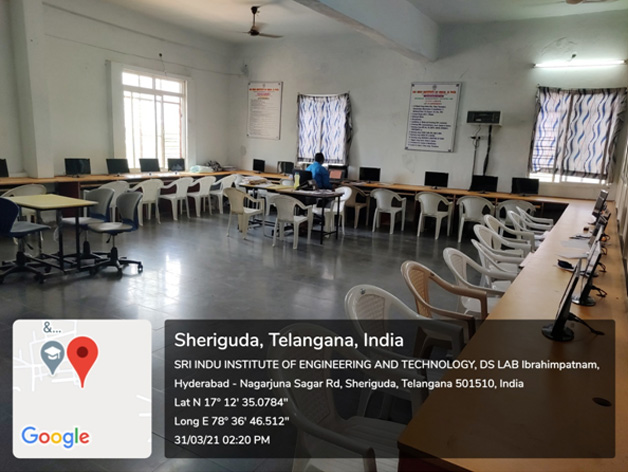
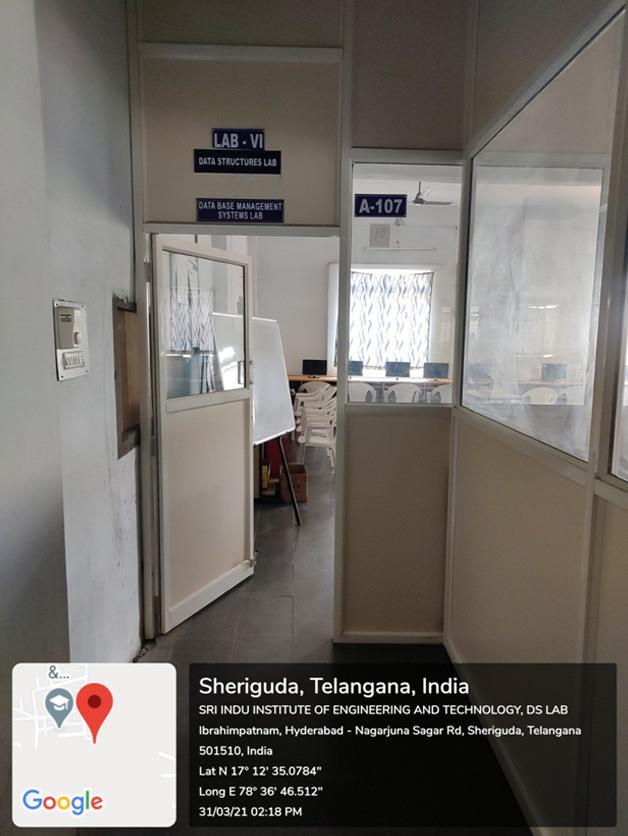
The objective of this laboratory is to link the theoretical concepts of different analog and digital electronics circuits with practical feasibility by giving to the students a scope to learn analog circuits and digital circuits in a better way.
The lab is well equipped with analog and digital electronic components, so students can fabricate their own circuit. The lab also comprises of Analog and digital trainer kits so as to facilitate verification of the results obtained through the fabricated circuits.
List of Experiments
- Full Wave Rectifier with & without filters
- Common Emitter Amplifier Characteristics
- Common Base Amplifier Characteristics
- Common Source amplifier Characteristics
- Measurement of h-parameters of transistor in CB, CE, CC configurations
- Input and Output characteristics of FET in CS configuration
- Realization of Boolean Expressions using Gates
- Design and realization logic gates using universal gates
- Generation of clock using NAND / NOR gates
- Design a 4 – bit Adder / Subtractor
- Design and realization a Synchronous and Asynchronous counter using flip-flops
- Realization of logic gates using DTL, TTL, ECL, etc.
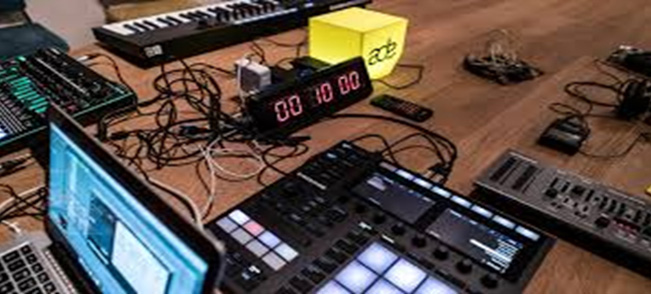
- Practical exposure on implementation of well known data mining tasks.
- Exposure to real life data sets for analysis and prediction.
- Learning performance evaluation of data mining algorithms in a supervised and an unsupervised setting.
- Handling a small data mining project for a given practical domain.
Task 1: Credit Risk Assessment
Description
The business of banks is making loans. Assessing the credit worthiness of an applicant is of crucial importance. You have to develop a system to help a loan officer decide whether the credit of a customer is good, or bad. A bank's business rules regarding loans must consider two opposing factors. On the one hand, a bank wants to make as many loans as possible. Interest on these loans is the banks profit source. On the other hand, a bank cannot afford to make too many bad loans. Too many bad loans could lead to the collapse of the bank. The bank's loan policy must involve a compromise: not too strict, and not too lenient.
To do the assignment, you first and foremost need some knowledge about the world of credit. You can acquire such knowledge in a number of ways.
- Knowledge Engineering. Find a loan officer who is willing to talk. Interview her and Try to represent her knowledge in the form of production rules.
- Books. Find some training manuals for loan officers or perhaps a suitable textbook on finance. Translate this knowledge from text form to production rule form.
- Common sense. Imagine yourself as a loan officer and make up reasonable rules Which can be used to judge the credit worthiness of a loan applicant.
- Case histories. Find records of actual cases where competent loan officers correctly judged when, and when not to, approve a loan application.
The German Credit Data
Actual historical credit data is not always easy to come by because of confidentiality rules. Here is one such dataset, consisting of 1000 actual cases collected in Germany. Credit dataset (original) Excel spreadsheet version of the German credit data.
In spite of the fact that the data is German, you should probably make use of it for this assignment. (Unless you really can consult a real loan officer!)
A few notes on the German dataset
- DM stands for Deutsche Mark, the unit of currency, worth about 90 cents Canadian (but looks and acts like a quarter).
- Owns telephone. German phone rates are much higher than in Canada so fewer people own telephones.
- Foreign worker. There are millions of these in Germany (many from Turkey). It is very hard to get German citizenship if you were not born of German parents.
- There are 20 attributes used in judging a loan applicant. The goal is to classify the applicant into one of two categories, good or bad.
Subtasks: (Turn in your answers to the following tasks)
- List all the categorical (or nominal) attributes and the real-valued attributes seperately. (5 marks)
- What attributes do you think might be crucial in making the credit assessment? Come up with some simple rules in plain English using your selected attributes. (5 marks)
- One type of model that you can create is a Decision Tree - train a Decision Tree using the complete dataset as the training data. Report the model obtained after training. (10 marks)
- Suppose you use your above model trained on the complete dataset, and classify credit good/bad for each of the examples in the dataset. What % of examples can you classify correctly? (This is also called testing on the training set) Why do you think you cannot get 100 % training accuracy? (10 marks)
- Is testing on the training set as you did above a good idea? Why or Why not ? (10 marks)
- One approach for solving the problem encountered in the previous question is using cross-validation? Describe what is cross-validation briefly. Train a Decision Tree again using cross validation and report your results. Does your accuracy increase/decrease? Why? (10 marks)
- Check to see if the data shows a bias against "foreign workers" (attribute 20), or "personal-status" (attribute 9). One way to do this (perhaps rather simple minded) is to remove these attributes from the dataset and see if the decision tree created in those cases is significantly different from the full dataset case which you have already done. To remove an attribute, you can use the preprocess tab in Weka's GUI Explorer. Did removing these attributes have any significant effect? Discuss. (10 marks)
- Another question might be, do you really need to input so many attributes to get good results? Maybe only a few would do. For example, you could try just having attributes 2, 3, 5, 7, 10, 17 (and 21, the class attribute (naturally)). Try out some combinations. (You had removed two attributes in problem 7. Remember to reload the arff data file to get all the attributes initially before you start selecting the ones you want.) (10 marks)
- Sometimes, the cost of rejecting an applicant who actually has a good credit (case 1) might be higher than accepting an applicant who has bad credit (case 2). Instead of counting the misclassifcations equally in both cases, give a higher cost to the first case (say cost 5) and lower cost to the second case. You can do this by using a cost matrix in Weka. Train your Decision Tree again and report the Decision Tree and crossvalidation results. Are they significantly different from results obtained in problem 6 (using equal cost)? (10 marks)
- Do you think it is a good idea to prefer simple decision trees instead of having long complex decision trees? How does the complexity of a Decision Tree relate to the bias of the model? (10 marks)
- You can make your Decision Trees simpler by pruning the nodes. One approach is to use Reduced Error Pruning - Explain this idea briefly. Try reduced error pruning for training your Decision Trees using cross-validation (you can do this in Weka) and report the Decision Tree you obtain? Also, report your accuracy using the pruned model. Does your accuracy increase? (10 marks)
- (Extra Credit): How can you convert a Decision Trees into "if-then-else rules". Makeup your own small Decision Tree consisting of 2-3 levels and convert it into a set of rules. There also exist different classifiers that output the model in the form of rules -one such classifier in Weka is rules. PART, train this model and report the set of rulesm obtained. Sometimes just one attribute can be good enough in making the decision, yes, just one ! Can you predict what attribute that might be in this dataset ? OneR classifier uses a single attribute to make decisions (it chooses the attribute based on minimum error). Report the rule obtained by training a one R classifier. Rank the performance of j48, PART and oneR. (10 marks)
Task Resources
- Mentor lecture on Decision Trees
- Andrew Moore's Data Mining Tutorials (See tutorials on Decision Trees and Cross Validation)
- Decision Trees (Source: Tan, MSU)
- Tom Mitchell's book slides (See slides on Concept Learning and Decision Trees)
- Weka resources
- Introduction to Weka (html version) (download ppt version)
- Download Weka
- Weka Tutorial
- ARFF format
- Using Weka from command line
Task 2: Hospital Management System
Data Warehouse consists Dimension Table and Fact Table.
REMEMBER The following
Dimension
The dimension object (Dimension):
- Name
- Attributes (Levels), with one primary key
- Hierarchies
About Levels and Hierarchies
Dimension objects (dimension) consist of a set of levels and a set of hierarchies defined over those levels. The levels represent levels of aggregation. Hierarchies describe parent-child relationships among a set of levels. For example, a typical calendar dimension could contain five levels. Two hierarchies can be defined on these levels:
H1: YearL > QuarterL > MonthL > WeekL > DayL
H2: YearL > WeekL > DayL
The hierarchies are described from parent to child, so that Year is the parent of Quarter, Quarter the parent of Month, and so forth.
About Unique Key Constraints
When you create a definition for a hierarchy, Warehouse Builder creates an identifier key for each level of the hierarchy and a unique key constraint on the lowest level (Base Level) Design a Hospital Management system data warehouse (TARGET) consists of Dimensions Patient, Medicine, Supplier, Time. Where measures are ‘NO UNITS’, UNIT PRICE.
Assume the Relational database (SOURCE) table schemas as follows
TIME (day, month, year),
PATIENT (patient_name, Age, Address, etc.,)
MEDICINE ( Medicine_Brand_name, Drug_name, Supplier, no_units, Uinit_Price, etc.,)
SUPPLIER :( Supplier_name, Medicine_Brand_name, Address, etc., )
If each Dimension has 6 levels, decide the levels and hierarchies, Assume the level names suitably.
Design the Hospital Management system data warehouse using all schemas. Give the example 4-D cube with assumption names.
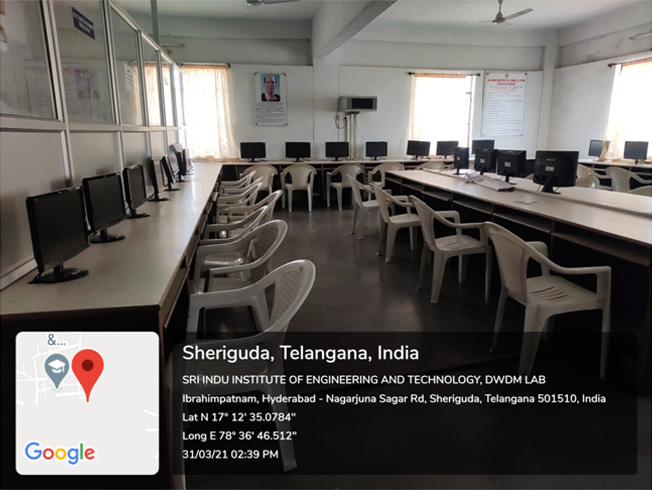
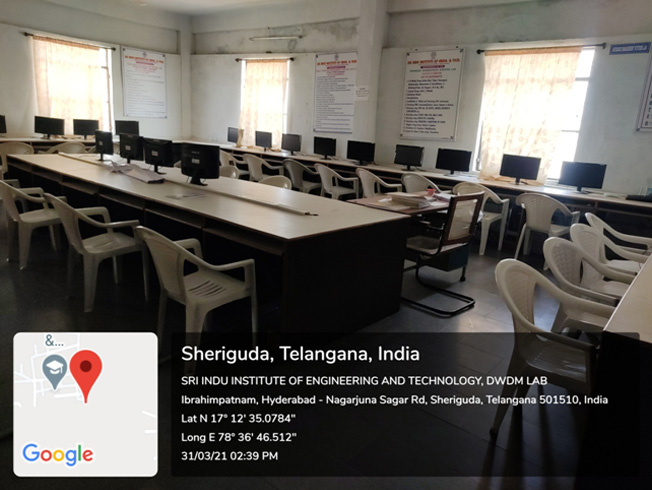

Objectives
- To understand basics of Cryptography and Network Security.
- To be able to secure a message over insecure channel by various means
- To learn about how to maintain the Confidentiality, Integrity and Availability of a data.
- To understand various protocols for network security to protect against the threats in the networks.
List of Experiments
- Write a C program that contains a string (char pointer) with a value ‘Hello world’. The program should XOR each character in this string with 0 and displays the result.
- Write a C program that contains a string (char pointer) with a value ‘Hello world’. The program should AND or and XOR each character in this string with 127 and display the result.
- Write a Java program to perform encryption and decryption using the following algorithms
a. Ceaser cipher b. Substitution cipher c. Hill Cipher - Write a C/JAVA program to implement the DES algorithm logic.
- Write a C/JAVA program to implement the Blowfish algorithm logic.
- Write a C/JAVA program to implement the Rijndael algorithm logic.
- Write the RC4 logic in Java Using Java cryptography; encrypt the text “Hello world” using Blowfish. Create your own key using Java key tool.
- Write a Java program to implement RSA algorithm.
- Implement the Diffie-Hellman Key Exchange mechanism using HTML and JavaScript.
- Calculate the message digest of a text using the SHA-1 algorithm in JAVA.
- Calculate the message digest of a text using the MD5 algorithm in JAVA.
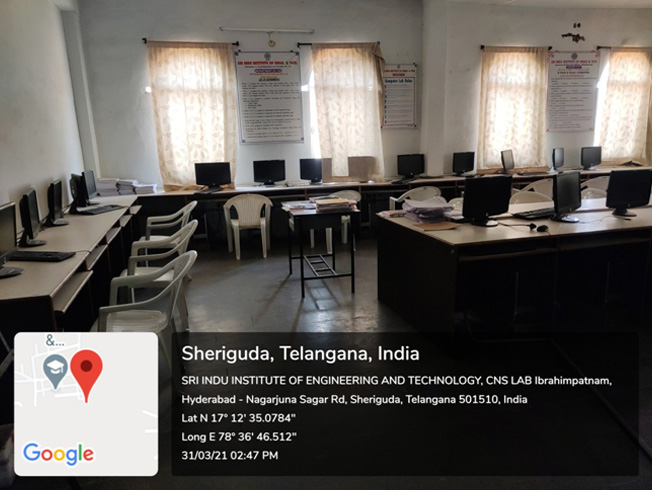
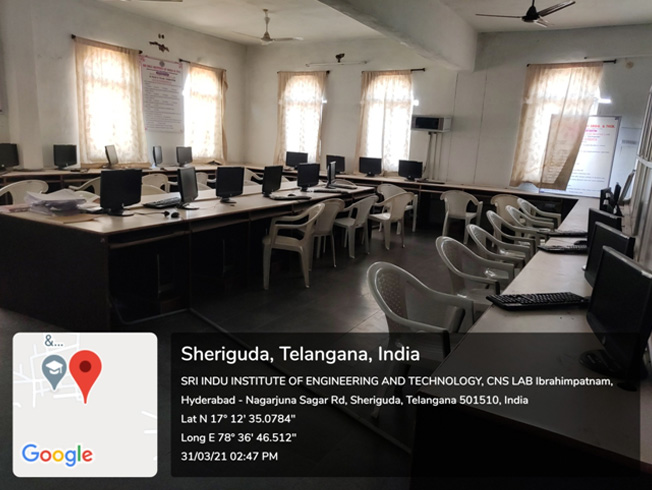
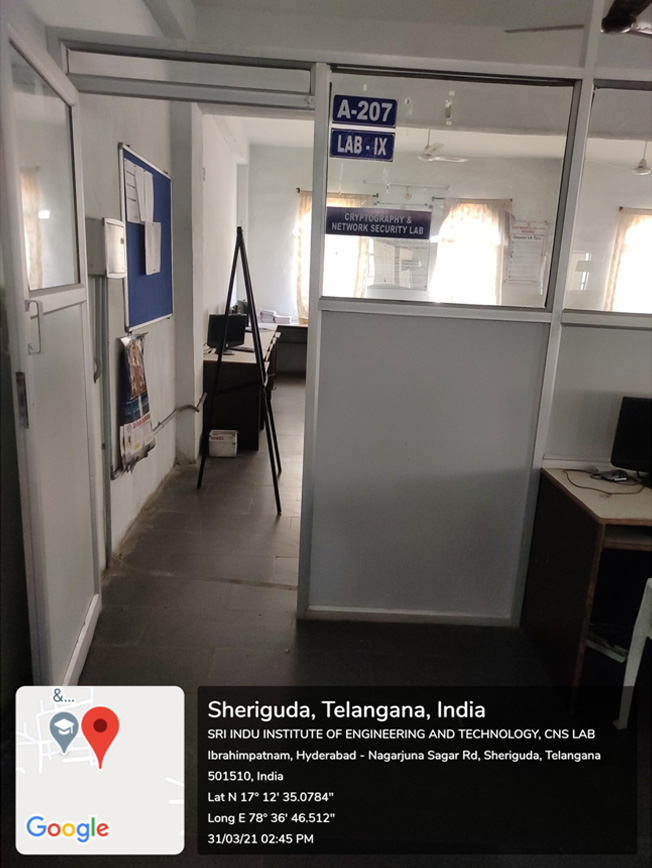
Objective
The IT Workshop for engineers is a 6 training lab course spread over 90 hours. The modules include training on PC Hardware, Internet, World Wide Web and Productivity tools including Word, Excel, PowerPoint and Publisher. PC Hardware introduces the students to a personal computer and its basic peripherals, the process of assembling a PC, installation of System Software MS-Windows, Linux and the required device drivers. In addition hardware and software level troubleshooting process, tips and tricks would be covered. Internet & WWW module introduces the different ways of hooking the PC on to the internet from home and workplace effectively usage of the internet. Usage of web browsers, e-mails, news groups and discussion forums would be covered. In addition, awareness of cyber hygiene, i.e., protecting the personal computer from getting infected with the viruses, worms and other cyber attacks would be introduced.
List of Experiments
PC Hardware
Task 1: Identify the peripherals of a computer, components in a CPU and its functions. Draw the block diagram of the CPU along with the configuration of each peripheral and submit to your instructor.
Task 2: Every student should disassemble and assemble the PC back to working condition. Lab instructors should verify the work and follow it up with a Viva. Also students need to go through the video which shows the process of assembling a PC. A video would be given as part of the course content.
Task 3: Every student should individually install MS windows on the personal computer. Lab instructor should verify the installation and follow it up with a Viva.
Task 4: Every student should install Linux on the computer. This computer should have windows installed. The system should be configured as dual boot with both windows and Linux. Lab instructors should verify the installation and follow it up with a Viva
Task 5: Hardware Troubleshooting: Students have to be given a PC which does not boot due to improper assembly or defective peripherals. They should identify the problem and fix it to get the computer back to working condition. The work done should be verified by the instructor and followed up with a Viva.
Task 6: Software Troubleshooting: Students have to be given a malfunctioning CPU due to system software problems. They should identify the problem and fix it to get the computer back to working condition. The work done should be verified by the instructor and followed up with a Viva.
Internet & World Wide Web
Task1: Orientation & Connectivity Boot Camp: Students should get connected to their Local Area Network and access the Internet. In the process they configure the TCP/IP setting. Finally students should demonstrate, to the instructor, how to access the websites and email. If there is no internet connectivity preparations need to be made by the instructors to simulate the WWW on the LAN.
Task 2: Web Browsers, Surfing the Web: Students customize their web browsers with the LAN proxy settings, bookmarks, search toolbars and pop up blockers. Also, plug-ins like Macromedia Flash and JRE for applets should be configured.
Task 3: Search Engines & Netiquette: Students should know what search engines are and how to use the search engines. A few topics would be given to the students for which they need to search on Google. This should be demonstrated to the instructors by the student.
Task 4: Cyber Hygiene: Students would be exposed to the various threats on the internet and would be asked to configure their computer to be safe on the internet. They need to first install an antivirus software, configure their personal firewall and windows update on their computer. Then they need to customize their browsers to block pop ups, block active x downloads to avoid viruses and/or worms.
LaTeX and WORD
Task 1 – Word Orientation: The mentor needs to give an overview of LaTeX and Microsoft (MS) office 2007/ equivalent (FOSS) tool word: Importance of LaTeX and MS office 2007/ equivalent (FOSS) tool Word as word Processors, Details of the four tasks and features that would be covered in each, Using LaTeX and word – Accessing, overview of toolbars, saving files, Using help and resources, rulers, format painter in word.
Task 2: Using LaTeX and Word to create project certificate. Features to be covered:- Formatting Fonts in word, Drop Cap in word, Applying Text effects, Using Character Spacing, Borders and Colors, Inserting Header and Footer, Using Date and Time option in both LaTeX and Word.
Task 3: Creating project abstract Features to be covered:-Formatting Styles, Inserting table, Bullets and Numbering, Changing Text Direction, Cell alignment, Footnote, Hyperlink, Symbols, Spell Check, Track Changes.
Task 4 : Creating a Newsletter : Features to be covered:- Table of Content, Newspaper columns, Images from files and clipart, Drawing toolbar and Word Art, Formatting Images, Textboxes, Paragraphs and Mail Merge in word.
Excel
Excel Orientation: The mentor needs to tell the importance of MS office 2007/ equivalent (FOSS) tool Excel as a Spreadsheet tool, give the details of the four tasks and features that would be covered in each. Using Excel – Accessing, overview of toolbars, saving excel files, Using help and resources.
Task 1: Creating a Scheduler - Features to be covered: Gridlines, Format Cells, Summation, auto fill, Formatting Text
Task 2: Calculating GPA - .Features to be covered:- Cell Referencing, Formulae in excel – average, std.deviation, Charts, Renaming and Inserting worksheets, Hyper linking, Count function, LOOKUP/VLOOKUP
Task 3: Performance Analysis - Features to be covered:- Split cells, freeze panes, group and outline, Sorting, Boolean and logical operators, Conditional formatting
LaTeX and MS/equivalent (FOSS) tool Power Point
Task 1: Students will be working on basic power point utilities and tools which help them create basic power point presentation. Topic covered during this week includes: - PPT Orientation, Slide Layouts, Inserting Text, Word Art, Formatting Text, Bullets and Numbering, Auto Shapes, Lines and Arrows in both LaTeX and PowerPoint. Students will be given model power point presentation which needs to be replicated (exactly how it’s asked).
Task 2: Second week helps students in making their presentations interactive. Topic covered during this week includes: Hyperlinks, Inserting –Images, Clip Art, Audio, Video, Objects, Tables and Charts.
Task 3: Concentrating on the in and out of Microsoft power point and presentations in LaTeX. Helps them learn best practices in designing and preparing power point presentation. Topic covered during this week includes: - Master Layouts (slide, template, and notes), Types of views (basic, presentation, slide slotter, notes etc), and Inserting – Background, textures, Design Templates, Hidden slides.
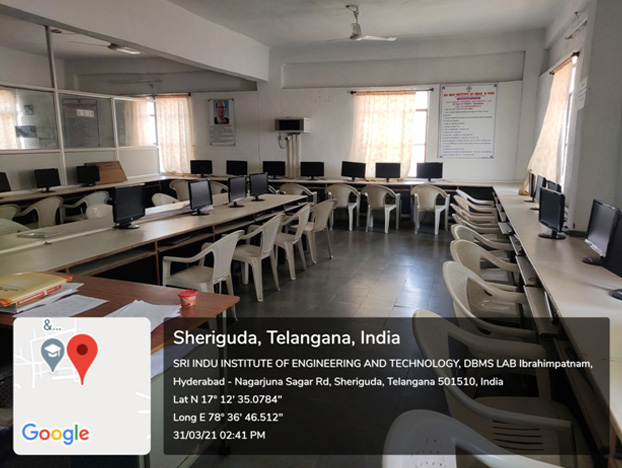
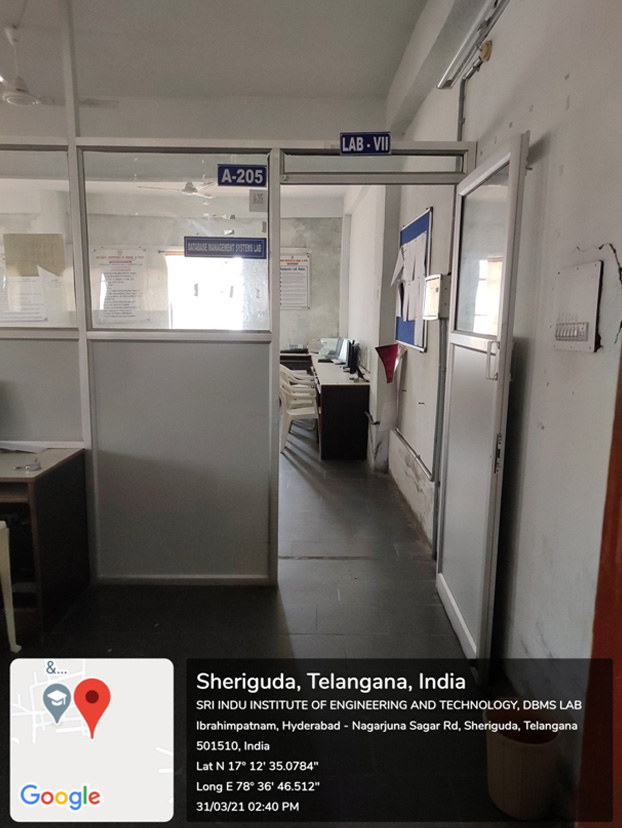
Labs for II Year II Semester
Program Objectives
- To provide an understanding of the design aspects of operating system concepts through simulation
- Introduce basic Unix commands, system call interface for process management, inter process communication and I/O in Unix
- Simulate and implement operating system concepts such as scheduling, deadlock management, file management and memory management
- Able to implement C programs using Unix system calls
List of Experiments
- Write C programs to simulate the following CPU Scheduling algorithms
a) FCFS b) SJF c) Round Robin d) priority - Write programs using the I/O system calls of UNIX/LINUX operating system (open, read, write, close, fcntl, seek, stat, opendir, readdir)
- Write a C program to simulate Bankers Algorithm for Deadlock Avoidance and Prevention.
- Write a C program to implement the Producer – Consumer problem using semaphores using UNIX/LINUX system calls.
- Write C programs to illustrate the following IPC mechanisms
a) Pipes b) FIFOs c) Message Queues d) Shared Memory - Write C programs to simulate the following memory management techniques
a) Paging b) Segmentation
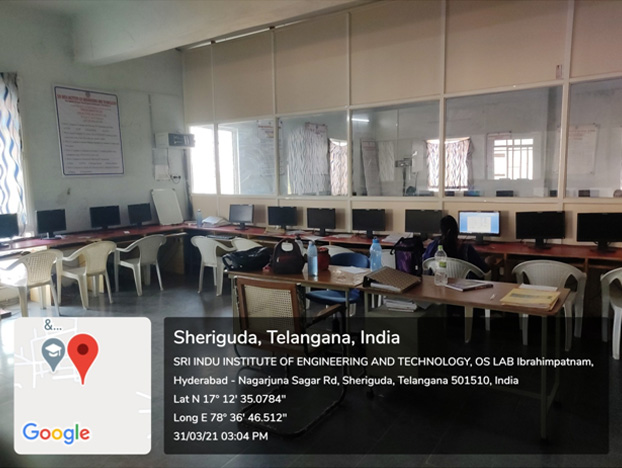
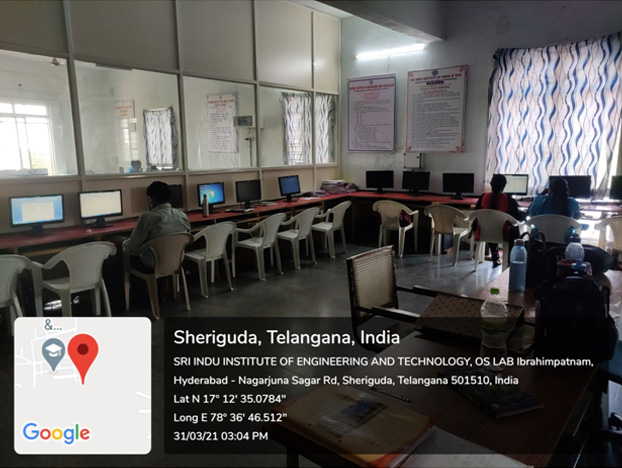
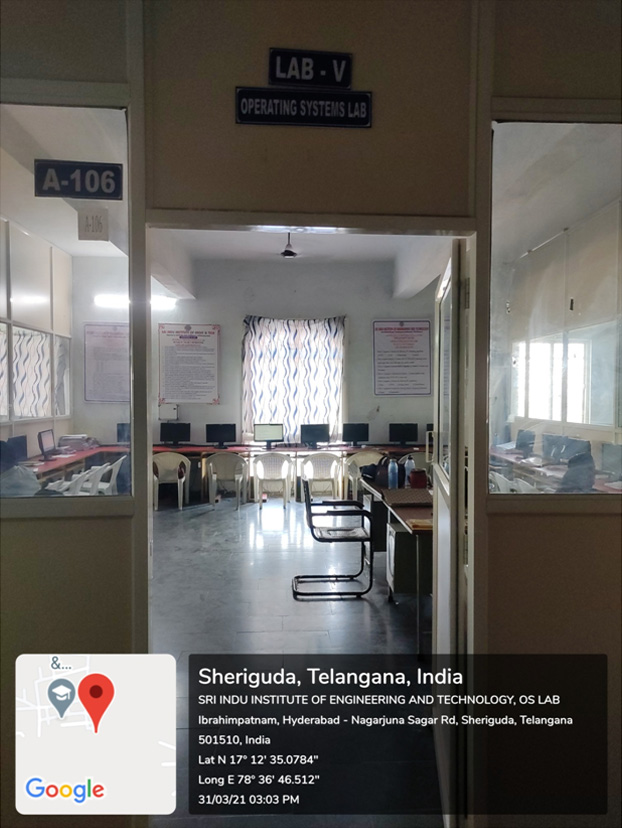
Objectives
Java Platform is a collection of programs that help programmers to develop and run Java programming applications efficiently. It includes an execution engine, a compiler, and a set of libraries in it. It is a set of computer software and specifications. James Gosling developed the Java platform at Sun Microsystems, and the Oracle Corporation later acquired it.
List of Experiments
- 1. Use Eclipse or Net bean platform and acquaint with the various menus. Create a test project, add a test class, and run it. See how you can use auto suggestions, auto fill. Try code formatter and code refactoring like renaming variables, methods, and classes. Try debug step by step with a small program of about 10 to 15 lines which contains at least one if else condition and a for loop.
- 2. Write a Java program that works as a simple calculator. Use a grid layout to arrange buttons for the digits and for the +, -,*, % operations. Add a text field to display the result. Handle any possible exceptions like divided by zero.
- a) Develop an applet in Java that displays a simple message.
b) Develop an applet in Java that receives an integer in one text field, and computes its Factorial Value and returns it in another text field, when the button named “Compute” is Clicked. - Write a Java program that creates a user interface to perform integer divisions. The user enters two numbers in the text fields, Num1 and Num2. The division of Num1 and Num 2 is displayed in the Result field when the Divide button is clicked. If Num1 or Num2 were not an integer, the program would throw a Number Format Exception. If Num2 were Zero, the program would throw an Arithmetic Exception. Display the exception in a message dialog box.
- Write a Java program that implements a multi-thread application that has three threads. First thread generates random integer every 1 second and if the value is even, second thread computes the square of the number and prints. If the value is odd, the third thread will print the value of cube of the number.
- Write a Java program for the following:
Create a doubly linked list of elements.
Delete a given element from the above list.
Display the contents of the list after deletion. - Write a Java program that simulates a traffic light. The program lets the user select one of three lights: red, yellow, or green with radio buttons. On selecting a button, an appropriate message with “Stop” or “Ready” or “Go” should appear above the buttons in selected color. Initially, there is no message shown.
- Write a Java program to create an abstract class named Shape that contains two integers and an empty method named print Area (). Provide three classes named Rectangle, Triangle, and Circle such that each one of the classes extends the class Shape. Each one of the classes contains only the method print Area () that prints the area of the given shape.
- Suppose that a table named Table.txt is stored in a text file. The first line in the file is the header, and the remaining lines correspond to rows in the table. The elements are separated by commas. Write a java program to display the table using Labels in Grid Layout
- Write a Java program that handles all mouse events and shows the event name at the center of the window when a mouse event is fired (Use Adapter classes).
- Write a Java program that loads names and phone numbers from a text file where the data is organized as one line per record and each field in a record are separated by a tab (\t). It takes a name or phone number as input and prints the corresponding other value from the hash table (hint:use hash tables).
- Write a Java program that correctly implements the producer – consumer problem using the concept of interthread communication.
- Write a Java program to list all the files in a directory including the files present in all its subdirectories.
- Write a Java program that implements Quick sort algorithm for sorting a list of names in Ascending order
- Write a Java program that implements Bubble sort algorithm for sorting in descending order and also shows the number of interchanges occurred for the given set of integers.
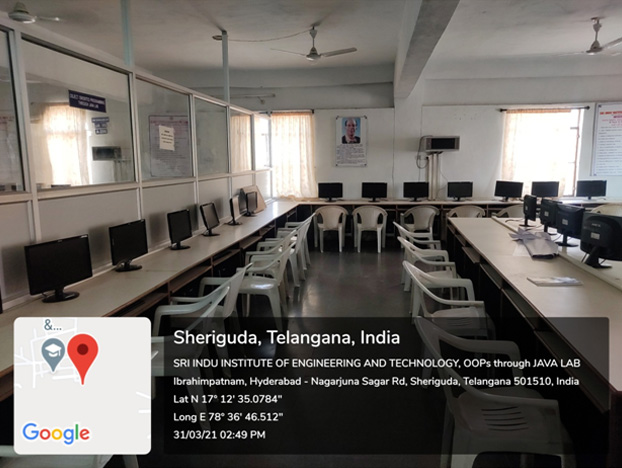
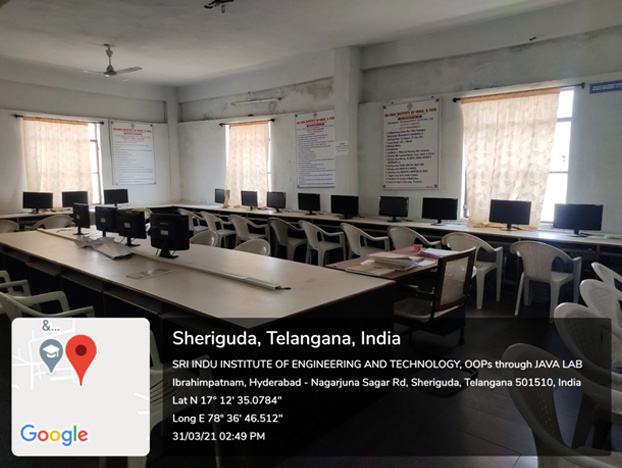
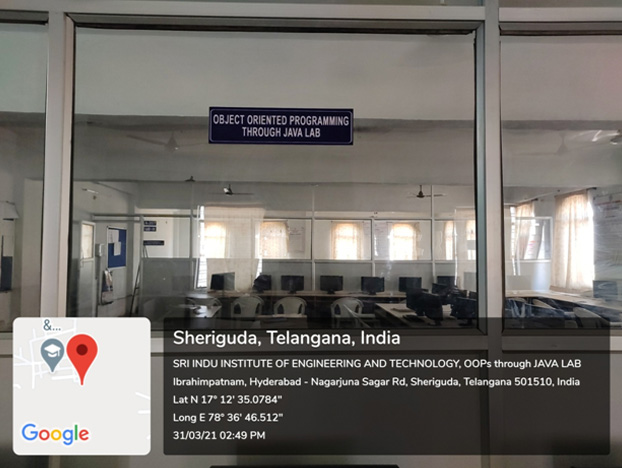
Database Management System (DBMS) is software for storing and retrieving users' data while considering appropriate security measures. It consists of a group of programs which manipulate the database. The DBMS accepts the request for data from an application and instructs the operating system to provide the specific data. In large systems, a DBMS helps users and other third-party software to store and retrieve data.
List of Experiments
- Concept design with E-R Model
- Relational Model
- Normalization
- Practicing DDL commands
- Practicing DML commands
- Querying (using ANY, ALL, IN, Exists, NOT EXISTS, UNION, INTERSECT, Constraints etc.)
- Queries using Aggregate functions, GROUP BY, HAVING and Creation and dropping of Views
- Triggers (Creation of insert trigger, delete trigger, update trigger)
- Procedures
- Usage of Cursors
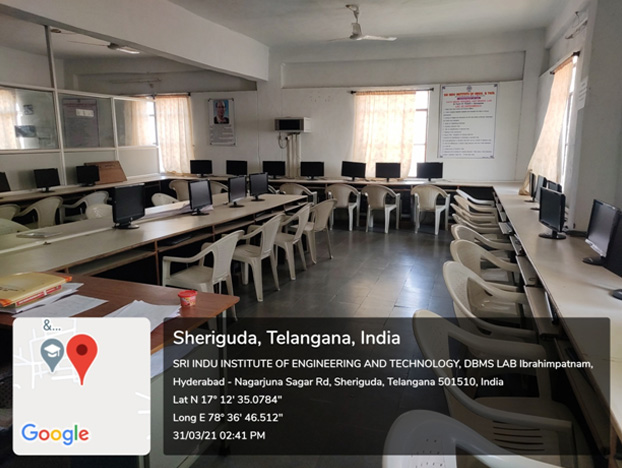
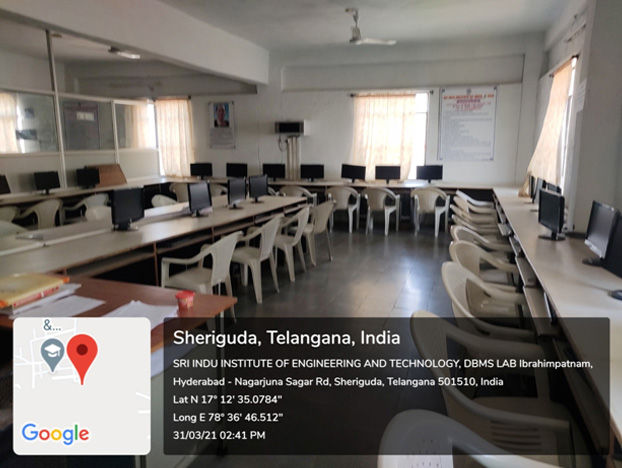
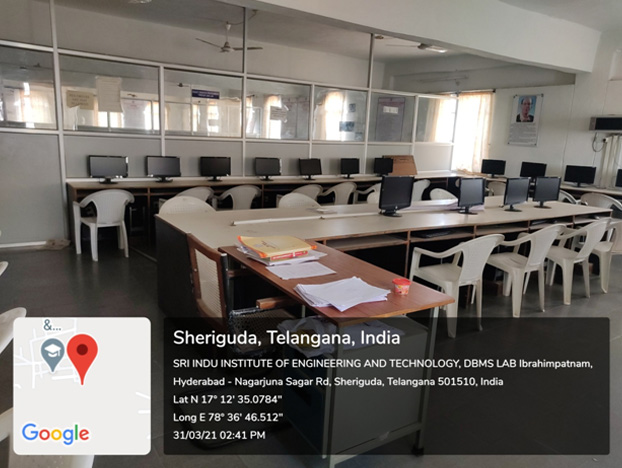
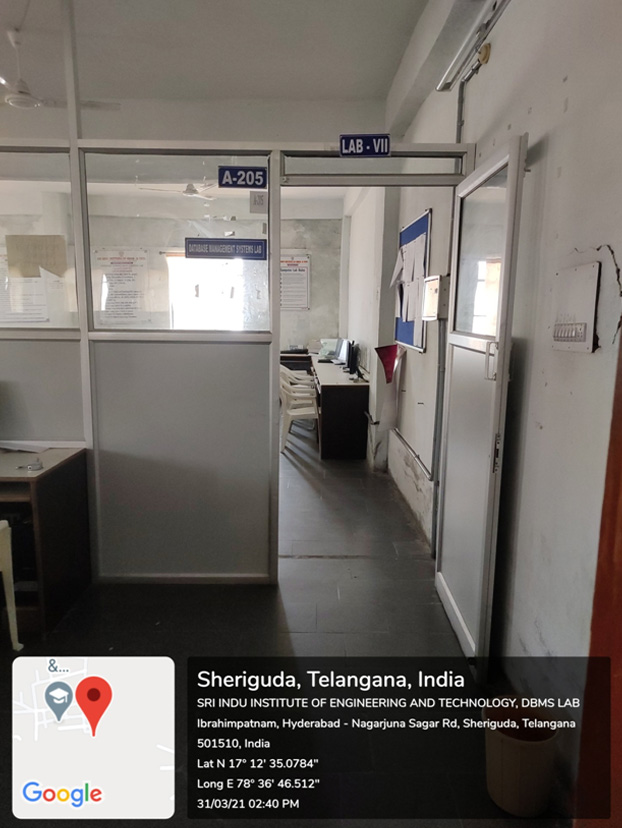
Labs for III Year I Semester
Objectives of the Lab
Maintainability – It should be feasible for the software to evolve to meet changing requirements. Efficiency – Correctness – Reusability – Testability – Reliability – Portability – Adaptability –
List of Experiments
- Development of problem statement
- Preparation of Software Requirement Specification Document, Design Documents and Testing Phase related documents.
- Preparation of Software Configuration Management and Risk Management related documents.
- Study and usage of any Design phase CASE tool
- Performing the Design by using any Design phase CASE tools.
- Develop test cases for unit testing and integration testing
- Develop test cases for various white box and black box testing techniques.
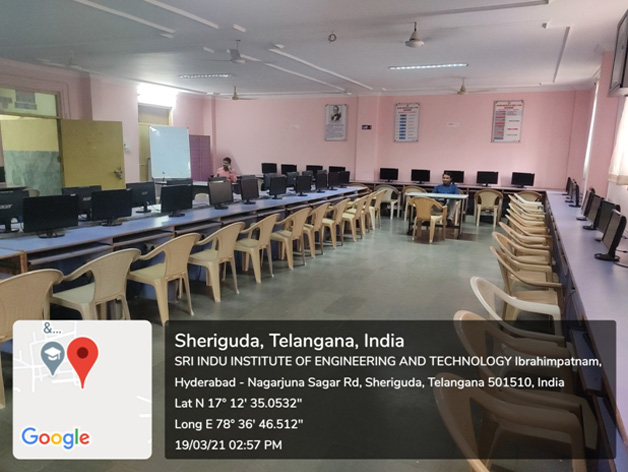
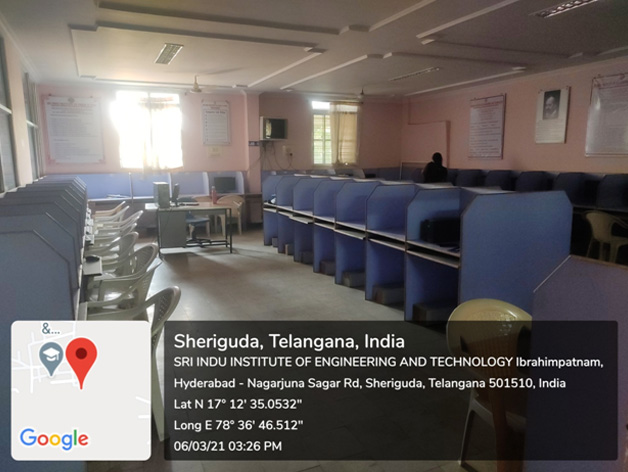
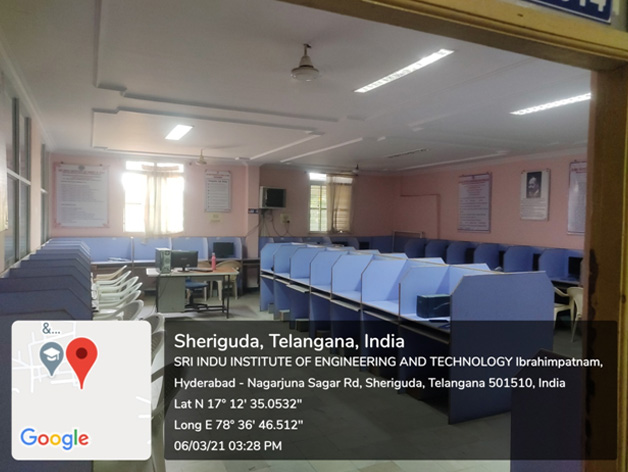
Objectives of the Lab
Determine the content of your message. Determine the best way to prepare and deliver your message verbally. Examine the basics of building a well-structured presentation. Examine the mechanics of delivering a successful presentation.
List of Experiments
Introduction
The introduction of the Advanced Communication Skills Lab is considered essential at 3rd year level. At this stage, the students need to prepare themselves for their careers which may require them to listen to, read, speak and write in English both for their professional and interpersonal communication in the globalized context.
The proposed course should be a laboratory course to enable students to use ‘good’ English and perform the following
- Gathering ideas and information to organize ideas relevantly and coherently
- Engaging in debates.
- Participating in group discussions
- Facing interviews.
- Writing project/research reports/technical reports.
- Making oral presentations.
- Writing formal letters.
- Transferring information from non-verbal to verbal texts and vice-versa.
- Taking part in social and professional communication.
Objectives
This Lab focuses on using multi-media instruction for language development to meet the following targets
- To improve the students’ fluency in English, through a well-developed vocabulary and enable them to listen to English spoken at normal conversational speed by educated English speakers and respond appropriately in different socio-cultural and professional contexts.
- Further, they would be required to communicate their ideas relevantly and coherently in writing.
- To prepare all the students for their placements.
Syllabus
The following course content to conduct the activities is prescribed for the Advanced English Communication Skills (AECS) Lab
- Activities on Fundamentals of Inter-personal Communication and Building Vocabulary - Starting a conversation – responding appropriately and relevantly – using the right body language – Role Play in different situations & Discourse Skills- using visuals - Synonyms and antonyms, word roots, one-word substitutes, prefixes and suffixes, study of word origin, business vocabulary, analogy, idioms and phrases, collocations & usage of vocabulary.
- Activities on Reading Comprehension – General Vs Local comprehension, reading for facts, guessing meanings from context, scanning, skimming, inferring meaning, critical reading& effective googling.
- Activities on Writing Skills – Structure and presentation of different types of writing – letter writing/Resume writing/ e-correspondence/Technical report writing/ – planning for writing – improving one’s writing.
- Activities on Presentation Skills – Oral presentations (individual and group) through JAM sessions/seminars/PPTs and written presentations through posters/projects/reports/ e- mails/assignments etc.
- Activities on Group Discussion and Interview Skills – Dynamics of group discussion, intervention, summarizing, modulation of voice, body language, relevance, fluency and organization of ideas and rubrics for evaluation- Concept and process, pre-interview planning, opening strategies, answering strategies, interview through tele-conference & video-conference and Mock Interviews.
Minimum Requirement
The Advanced English Communication Skills (AECS) Laboratory shall have the following infrastructural facilities to accommodate at least 35 students in the lab
- Spacious room with appropriate acoustics.
- Round Tables with movable chairs
- Audio-visual aids
- LCD Projector
- Public Address system
- P – IV Processor, Hard Disk – 80 GB, RAM–512 MB Minimum, Speed – 2.8 GHZ
- T. V, a digital stereo & Camcorder
- Headphones of High quality
Suggested Software
The software consisting of the prescribed topics elaborated above should be procured and used.
- Oxford Advanced Learner’s Compass, 7th Edition
- DELTA’s key to the Next Generation TOEFL Test: Advanced Skill Practice.
- Lingua TOEFL CBT Insider, by Dream tech
- TOEFL & GRE (KAPLAN, AARCO & BARRONS, USA, Cracking GRE by CLIFFS)
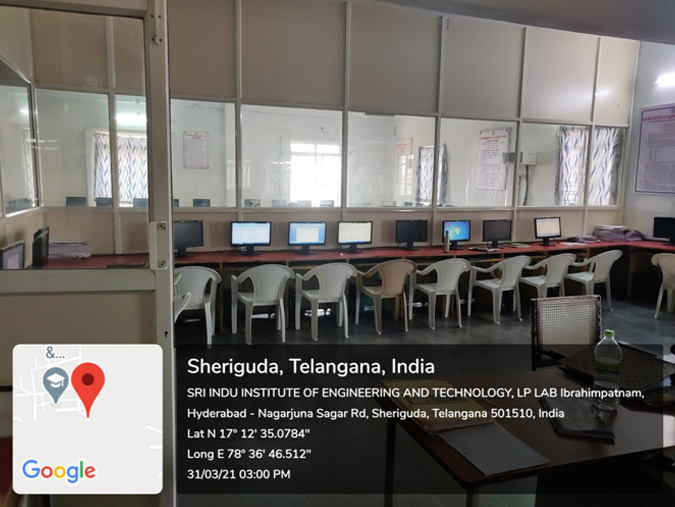
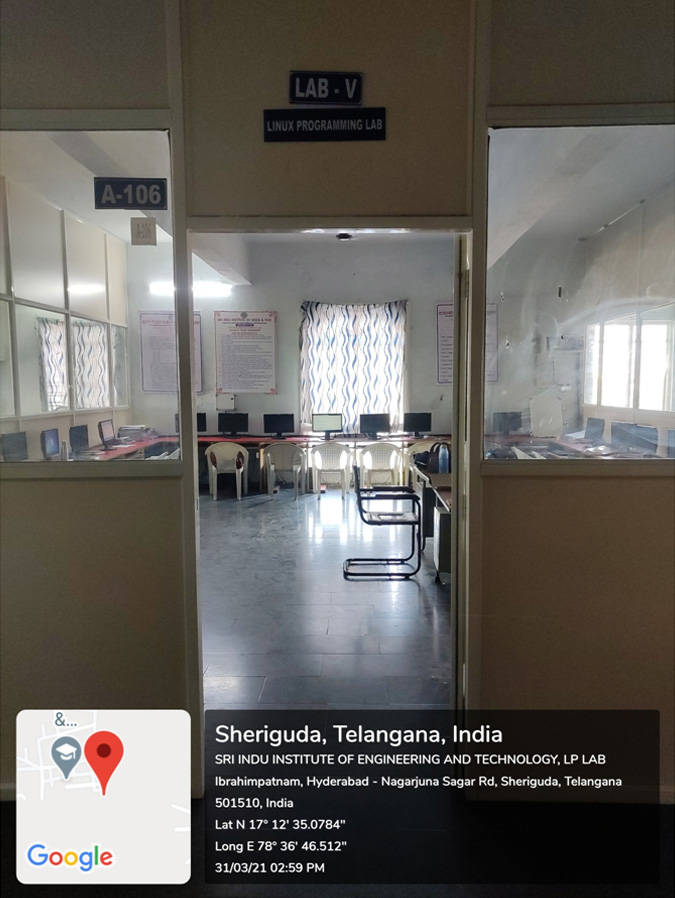
Labs for III Year II Semester
Objectives of the Lab
At the end of the course the students should be able to design and implement machine learning solutions to classification, regression, and clustering problems; and be able to evaluate and interpret the results of the algorithms.
List of Experiments
- The probability that it is Friday and that a student is absent is 3 %. Since there are 5 school days in a week, the probability that it is Friday is 20 %. What is theprobability that a student is absent given that today is Friday? Apply Baye’s rule in python to get the result. (Ans: 15%)
- Extract the data from database using python
- Implement k-nearest neighbours classification using python
- Given the following data, which specify classifications for nine combinations of VAR1 and VAR2 predict a classification for a case where VAR1=0.906 and VAR2=0.606, using the result of kmeans clustering with 3 means (i.e., 3 centroids) VAR1 VAR2 CLASS 1.713 1.586 0 0.180 1.786 1 0.353 1.240 1 0.940 1.566 0 1.486 0.759 1 1.266 1.106 0 1.540 0.419 1 0.459 1.799 1 0.773 0.186 1
- The following training examples map descriptions of individuals onto high, medium and low credit-worthiness. medium skiing design single twenties no -> highRisk high golf trading married forties yes -> lowRisk low speedway transport married thirties yes -> medRisk medium football banking single thirties yes -> lowRisk high flying media married fifties yes -> highRisk low football security single twenties no -> medRisk medium golf media single thirties yes -> medRisk medium golf transport married forties yes -> lowRisk high skiing banking single thirties yes -> highRisk low golf unemployed married forties yes -> highRisk Input attributes are (from left to right) income, recreation, job, status, age-group, home-owner. Find the unconditional probability of `golf' and the conditional probability of `single' given `medRisk' in the dataset?
- Implement linear regression using python
- Implement Naïve Bayes theorem to classify the English text
- Implement an algorithm to demonstrate the significance of genetic algorithm
- Implement the finite words classification system using Back-propagation algorithm
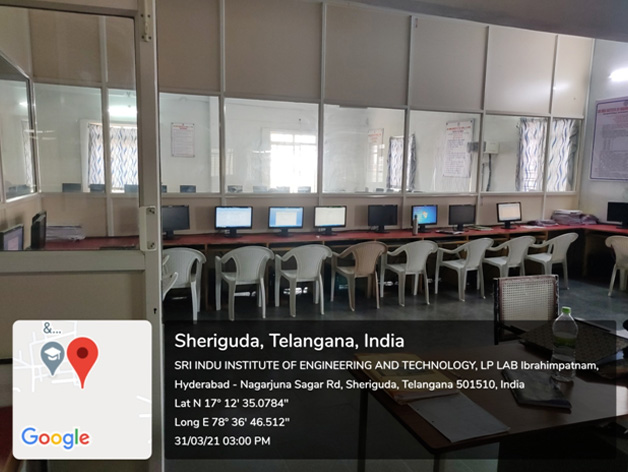
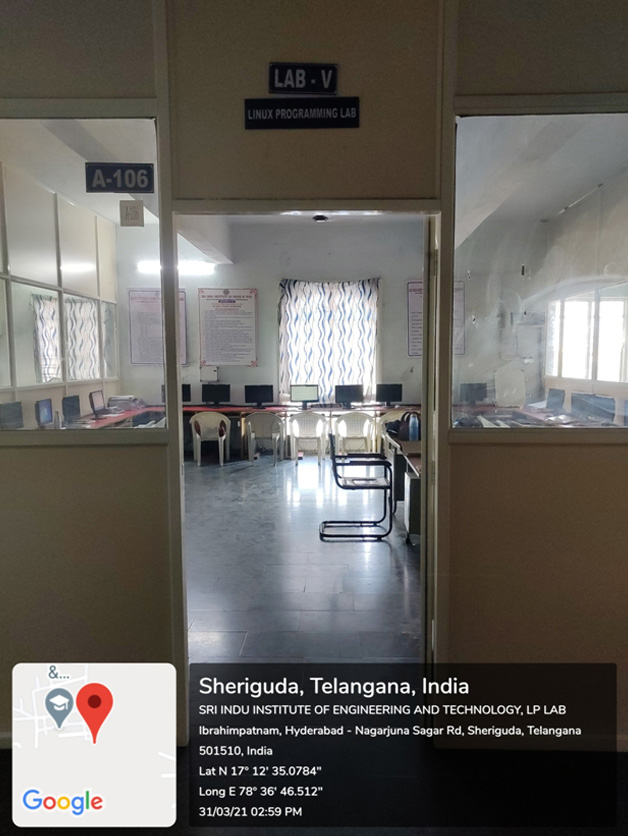
Objectives of the Lab
To design & implement a front end of the compiler. To develop program for implementing symbol table. To develop program for solving parser problems. To create program for intermediate code generation.
List of Experiments
- Write a LEX Program to scan reserved word & Identifiers of C Language
- Implement Predictive Parsing algorithm
- Write a C program to generate three address code
- Implement SLR(1) Parsing algorithm
- Design LALR bottom up parser for the given language ::= ::= { } | { } ::= int ; ::= | , ::= | [ ] ::= | ; ::= | | | | | ::= = | [ ] = ::= if then else endif | if then endif ::= while do enddo ::= print ( ) ::= | | ::= 114 ::= < | <= | == | >= | > | != ::= + | - ::= | ::= * | / ::= | | [ ] | ( ) ::= | ::= | ::= | ::= a|b|c|d|e|f|g|h|i|j|k|l|m|n|o|p|q|r|s|t|u|v|w|x|y|z ::= 0|1|2|3|4|5|6|7|8|9 has the obvious meaning Comments (zero or more characters enclosed between the standard C/Java-style comment brackets /*...*/) can be inserted. The language has rudimentary support for 1-dimensional arrays. The declaration int a[3] declares an array of three elements, referenced as a[0], a[1] and a[2]. Note also that you should worry about the scoping of names. A simple program written in this language is: { int a[3],t1,t2; t1=2; a[0]=1; a[1]=2; a[t1]=3; t2=-(a[2]+t1*6)/(a[2]-t1); if t2>5 then print(t2); else { int t3; t3=99; t2=-25; print(-t1+t2*t3); /* this is a comment on 2 lines */ } endif }
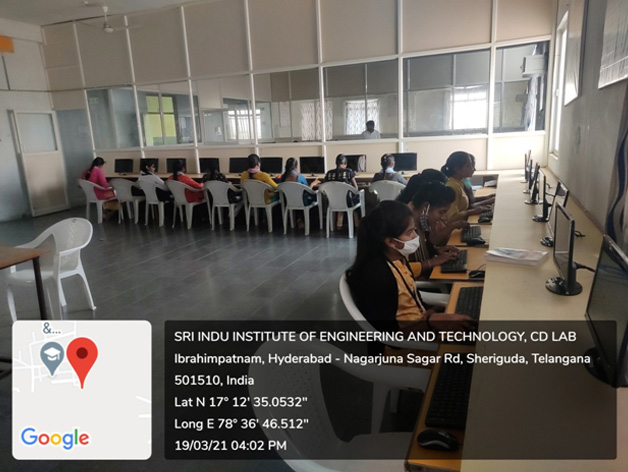
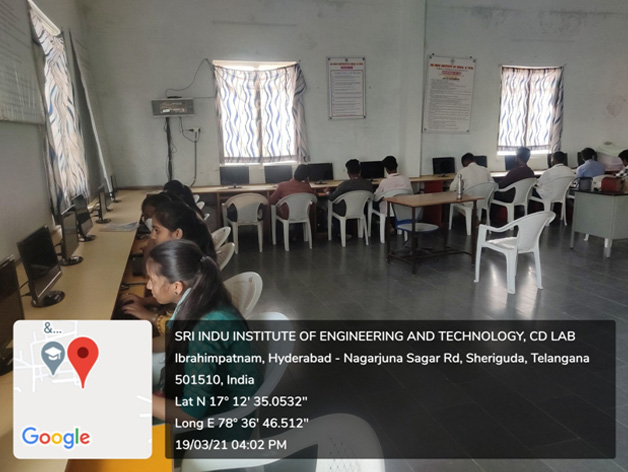
Objectives of the Lab
To provide knowledge of Software Testing Methods. To develop skills in software test automation and management using latest tools.
List of Experiments
- Recording in context sensitive mode and analog mode
- GUI checkpoint for single property
- GUI checkpoint for single object/window
- GUI checkpoint for multiple objects
- a) Bitmap checkpoint for object/window
b)Bitmap checkpoint for screen area - Database checkpoint for Default check
- Database checkpoint for custom check
- Database checkpoint for runtime record check
- a) Data driven test for dynamic test data submission
b) Data driven test through flat files
c) Data driven test through front grids
d) Data driven test through excel test - a) Batch testing without parameter passing
b) Batch testing with parameter passing
- Data driven batch
- Silent mode test execution without any interruption
- Test case for calculator in windows application
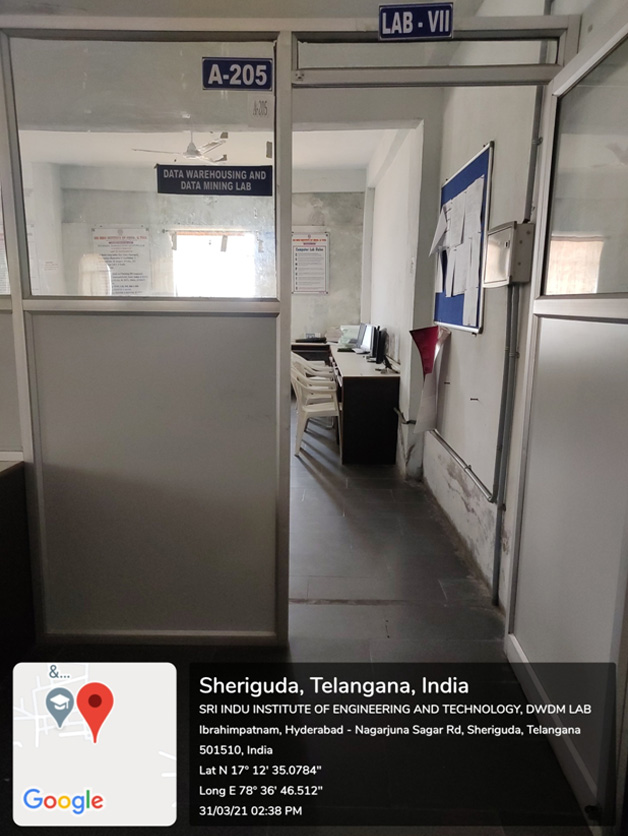
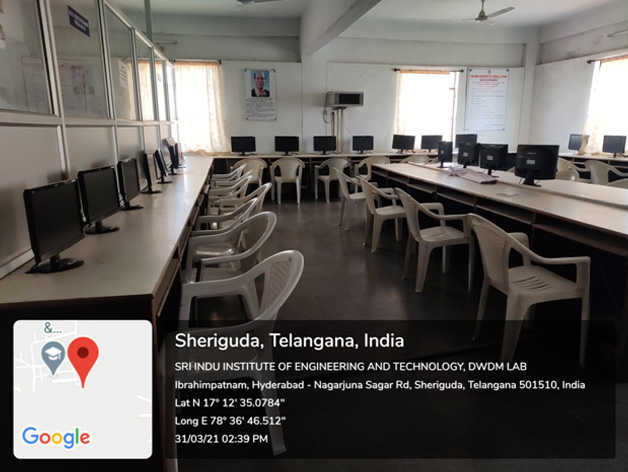
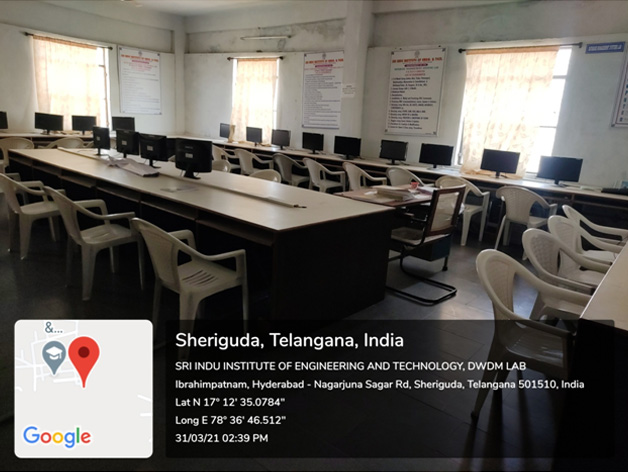
Labs for IV Year I Semester
Objectives of the Lab
- To learn and understand Python programming basics and paradigm.
- To learn and understand python looping, control statements and string manipulations.
List of Experiments
- Write a program to demonstrate different number data types in Python.
- Write a program to perform different Arithmetic Operations on numbers in Python.
- Write a program to create, concatenate and print a string and accessing sub-string from a given string.
- Write a python script to print the current date in the following format “Sun May 29 02:26:23 IST 2017”
- Write a program to create, append, and remove lists in python
- Write a program to demonstrate working with tuples in python.
- Write a program to demonstrate working with dictionaries in python.
- Write a python program to find largest of three numbers.
- Write a Python program to convert temperatures to and from Celsius, Fahrenheit. [Formula : c/5 = f-32/9 ]
- Write a Python program to construct the following pattern, using a nested for loop
- Write a Python program to construct the following pattern, using a nested for loop
- Write a python program to find factorial of a number using Recursion.
- Write a program that accepts the lengths of three sides of a triangle as inputs. The program output should indicate whether or not the triangle is a right triangle (Recall from the Pythagorean Theorem that in a right triangle, the square of one side equals the sum of the squares of the other two sides).
- Write a python program to define a module to find Fibonacci Numbers and import the module to another program.
- Write a python program to define a module and import a specific function in that module to another program.
- Write a script named copyfile.py. This script should prompt the user for the names of two text files. The contents of the first file should be input and written to the second file.
- Write a program that inputs a text file. The program should print all of the unique words in the file in alphabetical order.
- Write a Python class to convert an integer to a roman numeral.
- Write a Python class to implement pow(x, n)
- Write a Python class to reverse a string word by word.
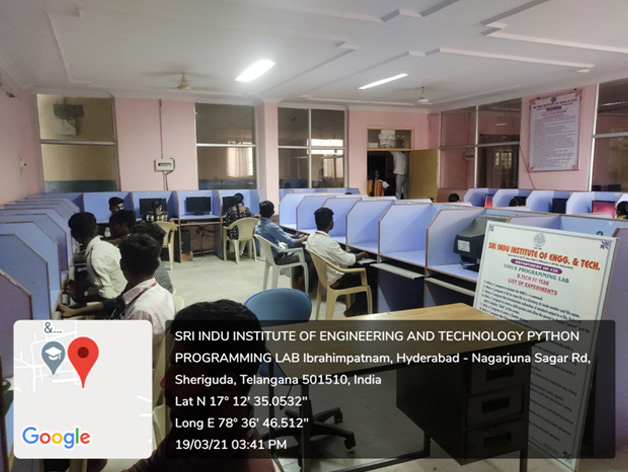
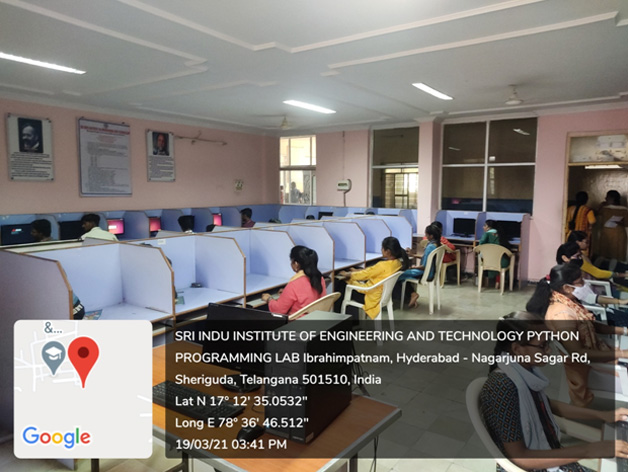
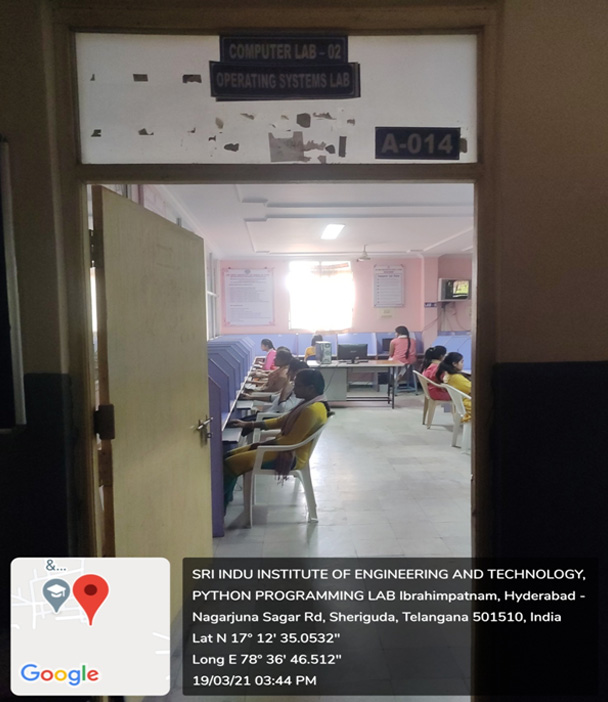